How to start a new Angular 9 Project in a few easy steps
In order to successfully create and run an Angular project locally, the following three software must be installed on your machine.
- VScode [Link]
Visual Studio Code is a source code editor developed by Microsoft for Windows, Linux, and macOS. It includes support for debugging, embedded Git control and GitHub, syntax highlighting, intelligent code completion, snippets, and code refactoring. - Node [Link]
Node.js is an open-source, cross-platform, JavaScript runtime environment that executes JavaScript code outside of a browser. - npm [Link]
npm is the world’s largest software registry. Open-source developers from every continent use npm to share and borrow packages and many organizations use npm to manage private development as well.
1 — Install Software
1.1 — Install Visual Studio Code
In order to write code, we must use an IDE, and Microsoft has developed an open-source and cross-platform IDE that can be run from anywhere regardless of which operating system you are using.
To install the IDE visit code.visualstudio.com and download the installation that best suits your case. Make sure to pick the stable version.

Once the software is downloaded to your local machine, run the package, and follow the instructions to complete the installation.
1.2 — Install Node and npm
Angular version 9 requires Node.js 10.13 at minimum to be installed. I recommend that you download the latest version (12.15.0 at the time this article was written).
To download Node.js visit nodejs.org/en/download and chose the installation package that matches your operating system.
I recommend that you chose the current version which will contain the latest features (if you are doing this for an enterprise project I recommend that you chose the Long Term Support package) when downloading the package.

Once the installation file is downloaded to your local machine, execute the installation and follow the steps. This package will also include and install npm.
Once the installation is complete you should be on your way to be able to start creating a shell Angular project.
To double check that the software were installed successfully you can do the following:
- Open Windows PowerShell or a Command Prompt
- Type in the following commands to check the version of the recently installed software:
c:\> node -v
c:\> npm -v
c:\> code -v - The versions of each installed software will be returned in the PowerShell. This will be an indication that they are successfully installed.
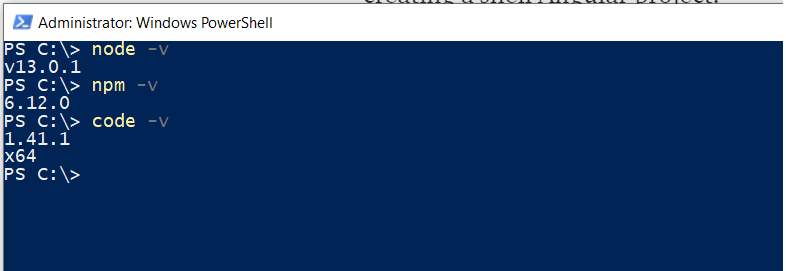
2 — Create Angular Project
In order to be able to easily create an angular project you must leverage the Angular CLI. The Angular CLI makes it easy to create an application that already works, right out of the box. The nice thing about using Angular CLI is that it follows the Angular best practices.
The Angular CLI is a command-line interface tool that you use to initialize, develop, scaffold, and maintain Angular applications.
2.1 — Install Angular CLI
There are many approaches to install the Angular CLI. In this tutorial we will install using the npm package manager within Visual Studio Code.
- Open a Windows PowerShell or a Command Prompt
- Create a new directory for the project. In this example I will create the project in its own directory located in the C drive.
C:\>mkdir angular-project - Navigate inside the newly created directory
C:\>cd angular-project - At the prompt type in the following command to open VScode
c:\>code . - Once VScode is launched, open a Terminal inside the IDE by either clicking the following combination: [ctrl +`] or from the navigation bar at the top of the IDE click on Terminal > New Terminal.
- In the terminal type the following command and press enter to begin installing the Angular CLI in our project root:
C:\angular-project> npm install -g @angular/cli@9

- You might be prompted to share anonymous usage data with the Angular Team at Google under Google’s Privacy Policy, I usually like to opt out. It is totally up to you. If you would like to share data, type y and hit enter. Otherwise type N.

NOTE: If you accidentally decided to share anonymous data and later on changed your mind you can always turn off analytics by typing in the following command:
C:\angular-project> ng analytics off
C:\angular-project> ng analytics off
- Now that the Angular CLI has been installed we are able to move on and create an Angular project from the command line interface.
2.2 — Create New Angular Project from CLI
To create a new project we will need to use the command ng new. This command creates and initializes a new Angular app that is the default project for a new workspace.
To learn more about the ng new you can refer to the Angular documentation: https://angular.io/cli/new
- In the terminal in your VScode IDE type in the following command (where shell-project is the name of your application/project) to create a project and click enter:
C:\angular-project> ng new shell-project - You will be prompted “Would you like to add Angular routing? (y/N)” and depending on your needs you might or might not need to install it.
You will definitely need to install it if you are going to offer routing in your application (this can always be added to the project in the future).
For this tutorial choose N and press enter

- Again you will be prompted with options to choose from for the stylesheet format that you would like to use in the project. Using your up and down arrow keys you will be able to make a selection.
For this tutorial choose the first option: CSS (.css) and press enter.

- The installation will take few minutes to complete and as soon as it is done installing you will receive a message stating: “√ Packages installed successfully.”

- Now that the project has been created we need to open it in VSCode. From VSCode top bar menu click on File > Open Folder and navigate to the angular-project and select the shell-project folder.

- Visual Studio Code will load the Angular project that we have just created. In the screen shot below the left pane of VScode displays the file structure.

- To run the project we need to use the following command in order to compile the code and launch it in a browser listening on a specific port (this port can be custom):
C:\angular-project\shell-project> ng serve --port 333 -o - In the command above we are telling angular to build the code and serve the application in a default browser while listening on port 333.
NOTE: In order to learn more about ng serve and the various options that it takes you can refer to the following documentation: https://angular.io/cli/serve

Below is a screen shot of the browser displaying the angular application that we have created. This page contains default information that is provided by Angular inside the app.component.html file and you will most likely want to replace it with something that suits you.

Now you have created your very first empty Angular 9 project and it is exciting to see what you will be able to do with it.
Comments
Post a Comment